Simplest way to Remove Ads via IAP
Published on: April 14, 2018
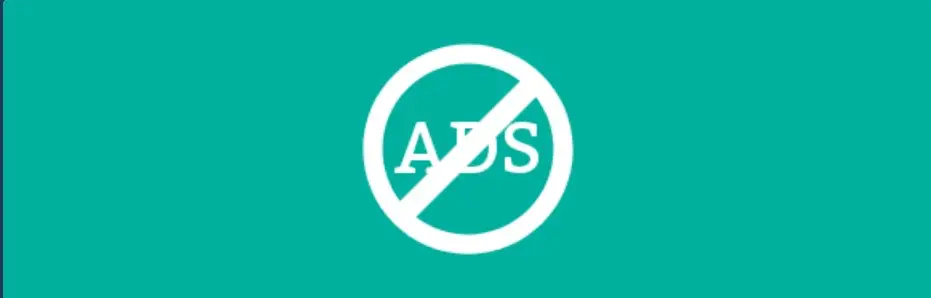
There are plenty of tutorials on the internet bout how to Remove Ads in your App via IAP on Android, but all are way harder & difficult for a beginner to understand. Someone with a little bit of Experience in Android will also find it a tad difficult.
In the beginning, even I faced problems with Android’s Own IAP library!
So here’s a simple tutorial for it.
This tutorial doesn't include parts like getting App’s API key & SKU (in app product name) from Play Developer Console.
Add the dependency as follows to grab the library –
groovycompile 'com.anjlab.android.iab.v3:library:+'
Next step, create a private variable like below in your
Activity
-javaprivate BillingProcessor mBillingProcessor;
Initialize the
BillingProcessor
in theonCreate()
like this –javamBillingProcessor = new BillingProcessor(MainActivity.this, "Your App’s API String Key here", new BillingProcessor.IBillingHandler() { @Override public void onProductPurchased(String productName, TransactionDetails transacDetails) { if (productName.equals("Product / SKU Name that you Created on Developer Console")) { relativeLayout.removeView(mAdView); } } @Override public void onBillingInitialized() {} @Override public void onPurchaseHistoryRestored() {} @Override public void onBillingError(int errorCode, Throwable throwable) {} });
What we did above was to Remove Ads when the payment is successful.
Now, init the library and remove ads if already purchased –
javamBillingProcessor.initialize(); if(mBillingProcessor.isPurchased("Your SKU Name")) relativelayout.removeView(mAdView);
You also need to override the
onActivityResult()
like this -java@Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { if (!mBillingProcessor.handleActivityResult(requestCode, resultCode, data)) { super.onActivityResult(requestCode, resultCode, data); } }
Simple, isn’t it!?
Okay, we have learnt how to handle AdView removal with purchase flow, but how do we start or call the Purchase?? Well, simply call below method and that’s it ✌️ –
javamBillingProcessor.purchase(MainActivity.this, "Your SKU Name");